# Titanium.UI.iOS.CollisionBehavior
Dynamic behavior to support collisions between items and boundaries.
# Overview
A collision behavior specifies the behavior when items collide with each other and boundaries. To define a collision behavior:
- Use the Titanium.UI.iOS.createCollisionBehavior method to create and define the behavior.
- Use the Titanium.UI.iOS.CollisionBehavior.addItem method to add items to the behavior.
- Use the Titanium.UI.iOS.CollisionBehavior.addBoundary method to add custom boundaries for the item to collide with. By default, the behavior uses the Animator object's reference view as the boundary.
- Add the behavior to an Titanium.UI.iOS.Animator.
# Examples
# Simple Example
The following example creates many blocks scattered across the top of the window, which start falling after the window opens. The item and boundary collisions are reported to the console.
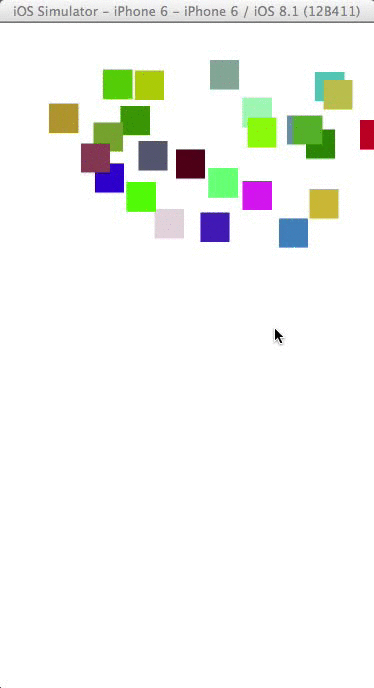
var win = Ti.UI.createWindow({backgroundColor: 'white', fullscreen: true});
// Create an Animator object using the window as the coordinate system
var animator = Ti.UI.iOS.createAnimator({referenceView: win});
// Create a default collision behavior, using the window edges as boundaries
var collision = Ti.UI.iOS.createCollisionBehavior();
// Listen for collisions
function report(e) {
Ti.API.info(JSON.stringify(e.type));
};
collision.addEventListener('itemcollision', report);
collision.addEventListener('boundarycollision', report);
// Simulate Earth's gravity
var gravity = Ti.UI.iOS.createGravityBehavior({
gravityDirection: {x: 0.0, y: 1.0}
});
var WIDTH = Ti.Platform.displayCaps.platformWidth;
var HEIGHT = Ti.Platform.displayCaps.platformHeight;
// Create a bunch of random blocks; add to the window and behaviors
var blocks = [];
for (var i = 0; i < 25; i++) {
var r = Math.round(Math.random() * 255);
var g = Math.round(Math.random() * 255);
var b = Math.round(Math.random() * 255);
var rgb = 'rgb(' + r +"," + g + "," + b + ")";
blocks[i] = Ti.UI.createView({
width: 25,
height: 25,
top: Math.round(Math.random() * (HEIGHT / 4) + 25),
left: Math.round(Math.random() * (WIDTH - 25) + 25),
backgroundColor: rgb
});
win.add(blocks[i]);
collision.addItem(blocks[i]);
gravity.addItem(blocks[i]);
}
animator.addBehavior(collision);
animator.addBehavior(gravity);
// Start the animation when the window opens
win.addEventListener('open', function(e){
animator.startAnimator();
});
win.open();
# Properties
# boundaryIdentifiers READONLY
Boundary identfiers added to this behavior.
# collisionMode
Specifies the collision behavior.
Default: Titanium.UI.iOS.COLLISION_MODE_ALL
# referenceInsets
Insets to apply when using the animator's reference view as the boundary.
The treatReferenceAsBoundary
property needs to be set to true
to use this property.
Default: All insets are zero.
# treatReferenceAsBoundary
Use the animator's reference view as the boundary.
Set to true
to enable this behavior or false
to disable it.
Default: true
# Methods
# addBoundary
Adds a boundary to this behavior.
Parameters
Name | Type | Description |
---|---|---|
boundary | BoundaryIdentifier | Boundary to add to the behavior. |
Returns
- Type
- void
# addItem
Adds an item to this behavior.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | View object to add to the behavior. |
Returns
- Type
- void
# removeAllBoundaries
Removes all boundaries from this behavior.
Returns
- Type
- void
# removeBoundary
Removes the specified boundary from this behavior.
Parameters
Name | Type | Description |
---|---|---|
boundary | BoundaryIdentifier | Boundary to remove. |
Returns
- Type
- void
# removeItem
Removes the specified item from this behavior.
Parameters
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item to remove. |
Returns
- Type
- void
# Events
# boundarycollision
Fired when an item collides with a boundary.
Properties
Name | Type | Description |
---|---|---|
item | Titanium.UI.View | Item that collided with the boundary. |
identifier | String | Identifier of the boundary the item collided with. |
point | Point | Point of the collision when it started. Only returned when |
start | Boolean | Returns |
source | Object | Source object that fired the event. |
type | String | Name of the event fired. |
bubbles | Boolean | True if the event will try to bubble up if possible. |
cancelBubble | Boolean | Set to true to stop the event from bubbling. |
# itemcollision
Fired when two items collide.
Properties
Name | Type | Description |
---|---|---|
item1 | Titanium.UI.View | Item that collided with |
item2 | Titanium.UI.View | Item that collided with |
point | Point | Point of the collision when it started. Only returned when |
start | Boolean | Returns |
source | Object | Source object that fired the event. |
type | String | Name of the event fired. |
bubbles | Boolean | True if the event will try to bubble up if possible. |
cancelBubble | Boolean | Set to true to stop the event from bubbling. |